How To Rename or Add Prefix to Multiple Files In Python (In Bulk)
Python is versatile programming language which is easy to learn for beginners. It’s usedIt’s working on on large scale projects as well as Small projects. Sometimes you want to rename or Add prefix to file name. However you can rename it easily by Right clicking on the file and Clicking on Rename. Let’s make twist, you want to add prefix to 100 files stored in specific folder. You are thinking about how long it will take to rename all the files.
Read More:
How To Install Python in Windows
This article will explain how to rename or add prefix into file name. We will use Python script to rename all the files stored in specific folder. It will also check for specific file extension.
How To Rename or Add Prefix Into the File Name Using Python Script
Python community serves thousands of libraries which helps in development. Python is dynamically typed and flexible, with code that is less verbose
Import libraries using below statements. argparse
to get user input from command line, os
to get list and rename all the files.
1
2
3
|
import os
import argparse
import sys
|
get_parser()
will get arguments from user and set them in variable.
1
2
3
4
5
6
|
def get_parser():
parser = argparse.ArgumentParser(description='Add prefix to files in a working directory')
parser.add_argument('work_dir', metavar='WORK_DIR', type=str, nargs=1, help='The directory where to add prefix')
parser.add_argument('ext', metavar='OLD_EXT', type=str, nargs=1, help='Extension')
parser.add_argument('prefix', metavar='NEW_EXT', type=str, nargs=1, help='Prefix')
return parser
|
Getting all the files from specific folder location and extension. After getting files rename()
will add prefix to fine name.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
|
def renameFiles(work_dir, old_ext, prefix):
print("Updating files: ")
files = os.listdir(work_dir)
for filename in files:
# Get the file extension
file_name = os.path.splitext(filename)[0]
file_ext = os.path.splitext(filename)[1]
# Check the file extensions
if old_ext == file_ext:
# Returns new file name having new prefix
newfile = prefix+file_name+file_ext
print(" " + filename)
# Rename File
os.rename(
os.path.join(work_dir, filename),
os.path.join(work_dir, newfile)
)
|
main()
Function To Call All The Actions
Getting array
of command line argument and calling the functions to rename all files.
1
2
3
4
5
6
7
|
def main():
parser = get_parser()
args = vars(parser.parse_args())
renameFiles(args['work_dir'][0], args['ext'][0], args['prefix'][0])
if __name__ == '__main__':
main()
|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
|
# How to Rename multiple files using python (In Bulk)
# Batch rename all the files in current directory
import os
import argparse
import sys
def renameFiles(work_dir, old_ext, prefix):
print("Updating files: ")
files = os.listdir(work_dir)
for filename in files:
# Get the file extension
file_name = os.path.splitext(filename)[0]
file_ext = os.path.splitext(filename)[1]
# Check the file extensions
if old_ext == file_ext:
# Returns new file name having new prefix
newfile = prefix+file_name+file_ext
print(" " + filename)
# Rename File
os.rename(
os.path.join(work_dir, filename),
os.path.join(work_dir, newfile)
)
def get_parser():
parser = argparse.ArgumentParser(description='Add prefix to files in a working directory')
parser.add_argument('work_dir', metavar='WORK_DIR', type=str, nargs=1, help='The directory where to add prefix')
parser.add_argument('ext', metavar='OLD_EXT', type=str, nargs=1, help='Extension')
parser.add_argument('prefix', metavar='NEW_EXT', type=str, nargs=1, help='Prefix')
return parser
def main():
parser = get_parser()
args = vars(parser.parse_args())
renameFiles(args['work_dir'][0], args['ext'][0], args['prefix'][0])
if __name__ == '__main__':
main()
|
Now open Command Prompt or Terminal and Run below command.
1
|
> py batch-file-rename.py f:/ .jpg myPrefix_
|
.jpg
: file extension
myPrefix_
: New prefix you want to add in file name.
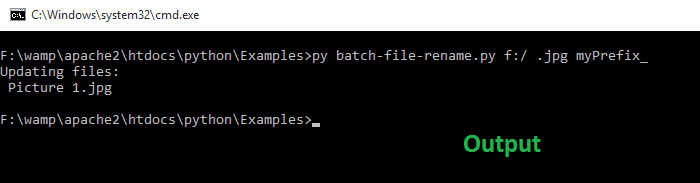
Output
Read More:
Create Twitter Bot Using Python and Tweepy
sSwitch – JQuery Plugin For Sliding Toggle Switches
How To Create Simple JQuery plugin
How To Implement Infinite Scroll Using JQuery, Ajax and PHP
Integrate Google Prettify in Html Page
Verify Your Server Meets PayPal Payment Gateway Requirements
Integrate google reCAPTCHA in your website
Continuing Education
Keep on writing, great job!
tulpan
I suggest to try KrojamSoft BatchRename